View previous topic :: View next topic |
Author |
Message |
kolusu Site Admin
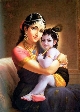
Joined: 26 Nov 2002 Posts: 12367 Topics: 75 Location: San Jose
|
Posted: Sun Dec 01, 2002 10:57 pm Post subject: Select top 3 salaries from Employee table |
|
|
Q.I have an 'employee' table with 3 columns emp-id, emp-name and emp-salary. I want to select first 3 employees in the order of their salary.The trick is to get the results without using a cursor and with a 'single' query.
Answer:
Code: |
SELECT * FROM EMP_TABLE
WHERE SAL >= (SELECT MAX(SAL) FROM EMP_TABLE
WHERE SAL < (SELECT MAX(SAL) FROM EMP_TABLE
WHERE SAL < (SELECT MAX(SAL)
FROM EMP_TABLE)))
ORDER BY SAL DESC
;
|
or
Code: |
SELECT * FROM
EMP_TABLE
WHERE
SAL = (SELECT MAX(SAL) FROM EMP_TABLE)
UNION
SELECT * FROM
EMP_TABLE
WHERE
SAL = (SELECT MAX(SAL) FROM EMP_TABLE
WHERE SAL < (SELECT MAX(SAL) FROM EMP_TABLE))
UNION
SELECT * FROM
EMP_TABLE
WHERE
SAL = (SELECT MAX(SAL) FROM EMP_TABLE
WHERE SAL < (SELECT MAX(SAL) FROM EMP_TABLE
WHERE SAL < (SELECT MAX(SAL) FROM EMP_TABLE)))
ORDER BY SAL DESC;
|
PS: UNION clause will remove any duplicates.If you want the duplicate values use UNION ALL
For a generic selection of first 'N' rows
Code: |
SELECT *
FROM
EMP_TABLE X
WHERE n > ( SELECT COUNT(*) FROM EMP_TABLE
WHERE SAL > X.SAL )
ORDER BY X.SAL DESC
;
|
Last edited by kolusu on Wed Oct 29, 2003 10:05 pm; edited 1 time in total |
|
Back to top |
|
 |
vallishar Beginner

Joined: 17 Dec 2002 Posts: 53 Topics: 14 Location: BengaLuru
|
Posted: Mon Feb 10, 2003 12:32 pm Post subject: |
|
|
Hi
Im doubtful of the order of execution in the first query. Could you pls explain? _________________ If you're not failing every now and again, it's a sign you're not doing anything very innovative. |
|
Back to top |
|
 |
gotluru Beginner
Joined: 17 Dec 2002 Posts: 29 Topics: 6
|
Posted: Mon Feb 10, 2003 2:18 pm Post subject: |
|
|
Hi Kolusu,
Did this select works for your question?
SELECT *
FROM
EMP_TABLE X
ORDER BY X.SAL DESC
FETCH FIRST 3 ROWS ONLY;
Prasad |
|
Back to top |
|
 |
vallishar Beginner

Joined: 17 Dec 2002 Posts: 53 Topics: 14 Location: BengaLuru
|
Posted: Mon Feb 10, 2003 2:50 pm Post subject: |
|
|
The Query with FIRST FETCH N ROWS gives the expected result too.
But the query
Quote: |
SELECT *
FROM
EMP_TABLE X
WHERE n > ( SELECT COUNT(*) FROM EMP_TABLE
WHERE SAL > X.SAL )
ORDER BY X.SAL DESC
;
|
Doesn't seem to be doing the same. _________________ If you're not failing every now and again, it's a sign you're not doing anything very innovative. |
|
Back to top |
|
 |
kolusu Site Admin
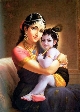
Joined: 26 Nov 2002 Posts: 12367 Topics: 75 Location: San Jose
|
Posted: Mon Feb 10, 2003 6:59 pm Post subject: |
|
|
vallish,
can you elaborate as to what you meant by "it doesn't seem to be the doing the same"?
Gotluru: your select statement with FETCH FIRST 3 ROWS also works.It is a new feature of db2 v7. Also it wouldn't elimintate duplicates.
Kolusu |
|
Back to top |
|
 |
vallishar Beginner

Joined: 17 Dec 2002 Posts: 53 Topics: 14 Location: BengaLuru
|
Posted: Tue Feb 11, 2003 10:26 am Post subject: |
|
|
Kolusu,
I tried to execute the SQL
Quote: |
SELECT *
FROM
EMP_TABLE X
WHERE n > ( SELECT COUNT(*) FROM EMP_TABLE
WHERE SAL > X.SAL )
ORDER BY X.SAL DESC
;
|
With the value for N = 3. The table contains 10 rows. This query is returning me all the 10 rows. Am I doing anything wrong? _________________ If you're not failing every now and again, it's a sign you're not doing anything very innovative. |
|
Back to top |
|
 |
kolusu Site Admin
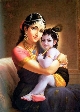
Joined: 26 Nov 2002 Posts: 12367 Topics: 75 Location: San Jose
|
Posted: Tue Feb 11, 2003 9:44 pm Post subject: |
|
|
vallishar,
The query works fine for me , can you post your sql so that I can take a look at it?
Thanks
kolusu |
|
Back to top |
|
 |
vallishar Beginner

Joined: 17 Dec 2002 Posts: 53 Topics: 14 Location: BengaLuru
|
Posted: Thu Feb 13, 2003 11:19 am Post subject: |
|
|
Hi Kolusu,
The query gives the correct result for the distinct values of the selected field., in this case emp_sal. When there are duplicate rows, the number of rows selected may not be the same as expected.
I had overlooked the result of my query. Aplogies.
Thanks _________________ If you're not failing every now and again, it's a sign you're not doing anything very innovative. |
|
Back to top |
|
 |
Premkumar Moderator

Joined: 28 Nov 2002 Posts: 77 Topics: 7 Location: Chennai, India
|
Posted: Thu Feb 13, 2003 11:48 pm Post subject: |
|
|
That is a query to select 'Top n, with ties for the last place' and NOT 'First n'. |
|
Back to top |
|
 |
Glenn Beginner
Joined: 23 Mar 2003 Posts: 56 Topics: 3
|
Posted: Sun Mar 23, 2003 7:32 am Post subject: |
|
|
Might I suggest?
Code: |
select a1.salary1, count(*)
from
(select e1.salary as salary1,
e2.salary as salary2
from employee e1,
employee e2
group by e1.salary, e2.salary) A1
where a1.salary1 <= a1.salary2
group by a1.salary1
order by a1.salary1 desc;
|
as a start? It produces something like:
Code: |
EMP_SALARY RANKING
============================
52750.00 1
46500.00 2
41250.00 3
40175.00 4
38250.00 5
36170.00 6
32250.00 7
29840.00 8
29750.00 9
29250.00 10
28760.00 11
28420.00 12
27740.00 13
27380.00 14
26250.00 15
26150.00 16
25370.00 17
25280.00 18
24680.00 19
23840.00 20
23800.00 21
22250.00 22
22180.00 23
21340.00 24
20450.00 25
19950.00 26
19180.00 27
18270.00 28
17750.00 29
17250.00 30
15900.00 31
15340.00 32
|
Edited - different statement which handles duplicates better |
|
Back to top |
|
 |
rama krishna reddy Beginner
Joined: 18 Sep 2006 Posts: 31 Topics: 13 Location: Hyderabad
|
Posted: Thu Apr 19, 2007 12:54 pm Post subject: MAX(SAL) |
|
|
Is there any other way to select the second or third max value in a column..apart from the below query.
select max(sal) from table where sal < (select max(sal) from table)
Hi Madhuri
We can select first max or third max whar ever u want by using below correlated query
select a.emplno ,a.salary from employee a where 0=(select count(*) from employee b where a.salary < b.salary)
In the above case it will give maximum salary ,if u want second max u need to give 1 inspite of 0 ,if u want third max u need to give 2 inspite of 0
eg:
i/p Employee table date is
500
400
200
300
100
query will sort the input table like below
In this case
a b
100 100
200 200
300 300
400 400
500 500
if u consider salary one in this case count will beocome 4 science
100 is less than 200,300,400,500
in case of 500 COUNT will be zero
I hope it will clear u r doubt
Regards
Rama krishna reddy |
|
Back to top |
|
 |
kolusu Site Admin
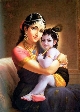
Joined: 26 Nov 2002 Posts: 12367 Topics: 75 Location: San Jose
|
|
Back to top |
|
 |
|
|